Vietnamese Cuisine Generator
Front-end Development | UI Design | Web-based Application
January - March 2023
Note: The site only works on desktop screens.
Vietnamese Cuisine Generator is a creative version of the traditional zodiac
chart. This is a fun “game” where users can find out their featured personality traits and which
Vietnamese food represents them by entering their birthdays.
This is also my first coding-heavy assignment in class. I have created a simple website with
HTML and CSS before, and this time I learned to implement JavaScript to design a dynamic and
interactive site with sounds and visual effects. Since this is an individual project, I took up
both design and development tasks.
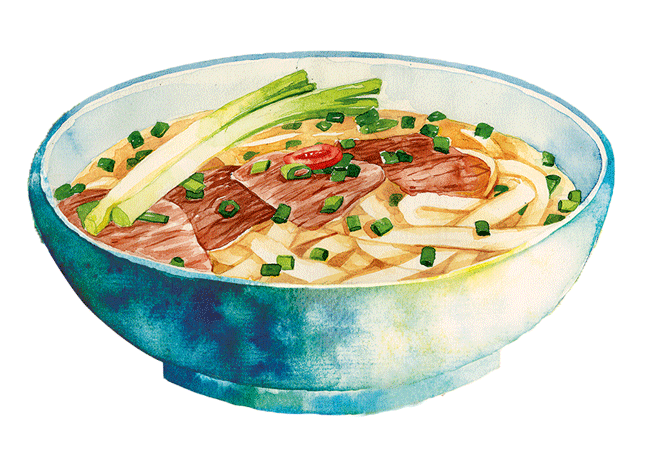
1. A simple but optimal design
I got started with the project by building the content and designing the interface as they are the backbones for the coding process.
The requirement of the assignment is to create an application based on Western Zodiac algorithm - I was given some creative freedom to apply a theme to the app. I chose Vietnamese cuisine as the theme - this is a part of my culture that I want to introduce to people. For the content, I collected food illustrations with similar design to maintain the consistency throughout the site, and also prioritized those with descriptive details to help users picture the dishes better. I wrote a short introduction under each dish’s name to provide some information about what kind of food it is, and what ingredients it has. This was a fun part of the project as I got a chance to learn some interesting facts about the foods that are familiar to me. Next, I assigned each dish a zodiac sign, either randomly or based on the “personality” that the dish has.
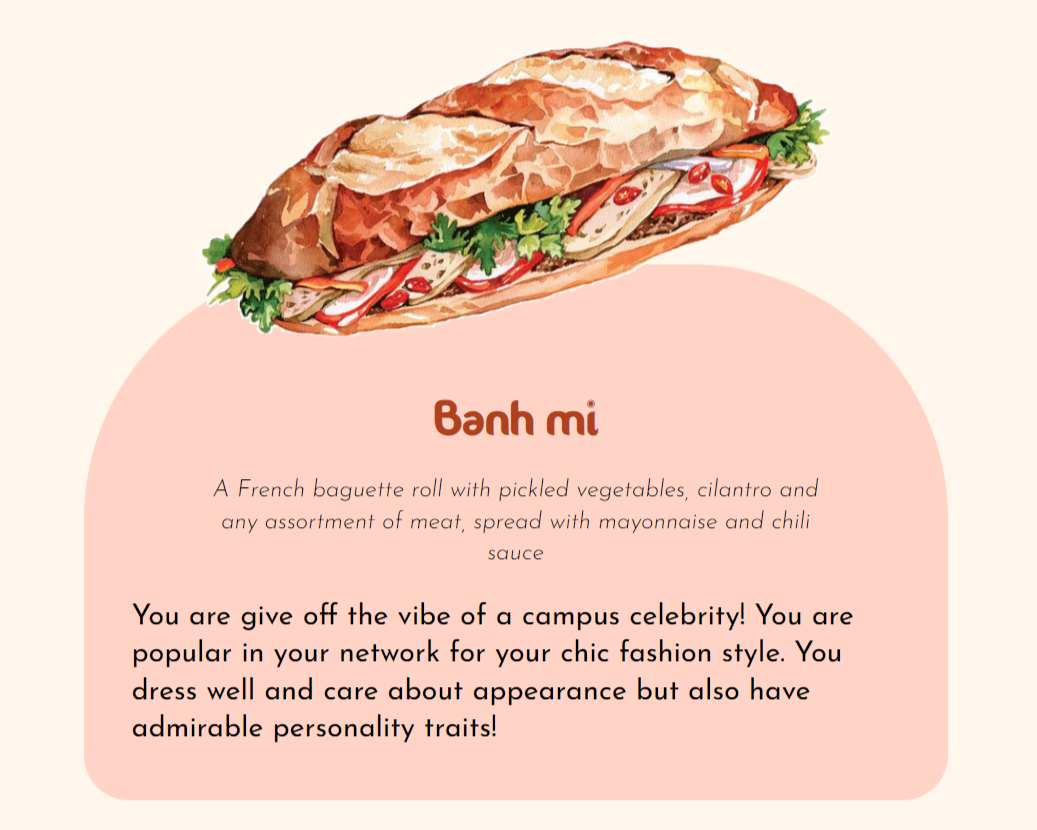
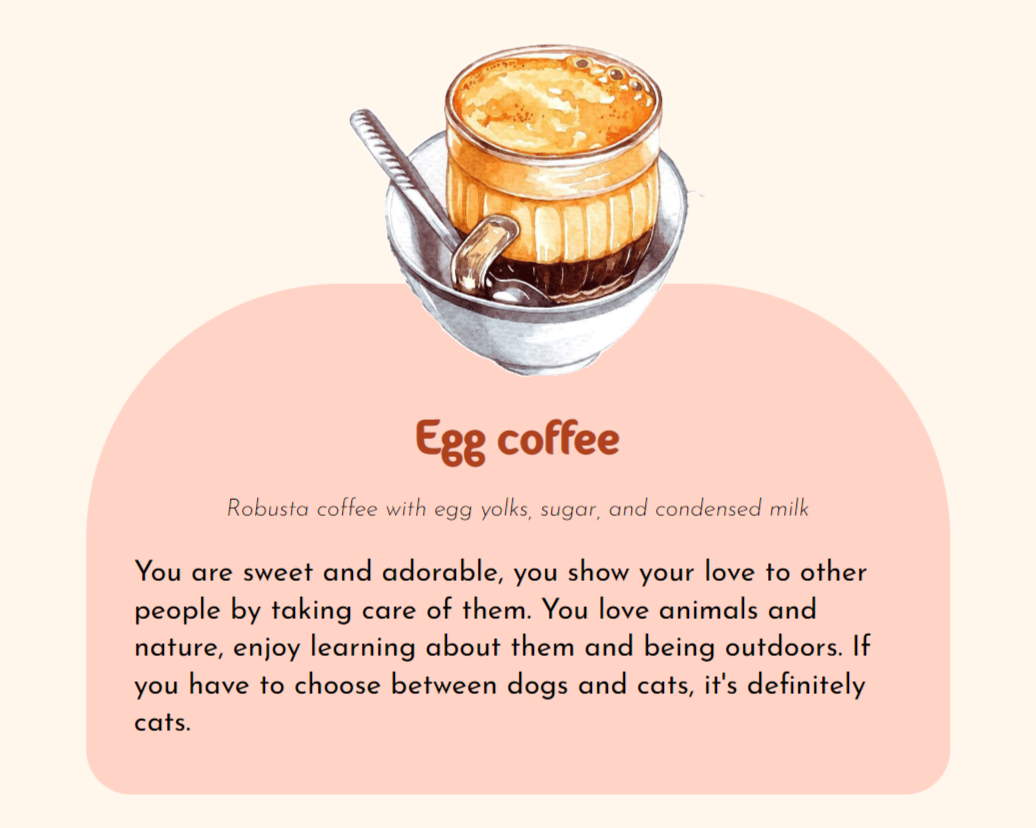
For the design process, I started with the color palette - my favorite part. Warm colors such as red, orange, and yellow immediately came to my mind as they are very representative of Vietnamese food. The green color was added to the palette at first since a feature of Vietnamese cuisine is that the foods always have a decent amount of vegetables. However, I decided to remove it because it’s a little tricky to incorporate this cool color with the warm ones, and an analogous color scheme is basic enough to make the design look coherent but not overpower the vivid colors of the illustrations. The layout of the site is clearly divided into 3 main columns - two “sub-menu” columns on the sides for users to explore other Vietnamese foods that don’t represent their signs, and a main column at the center with large-scale image and bold text to emphasize the dish description.
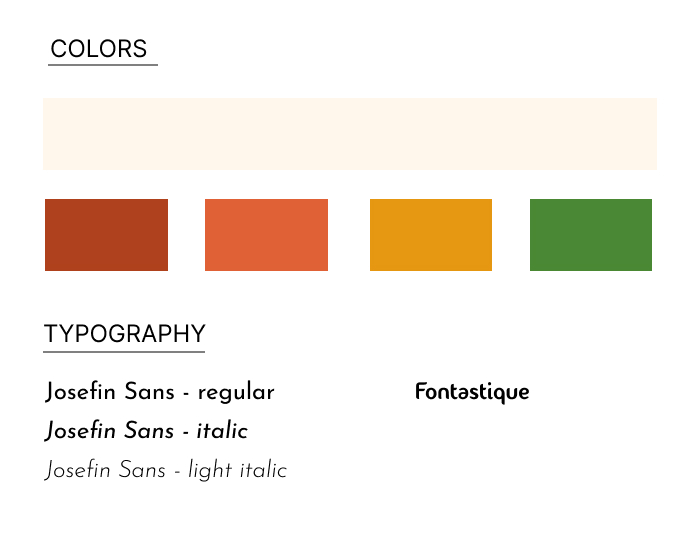
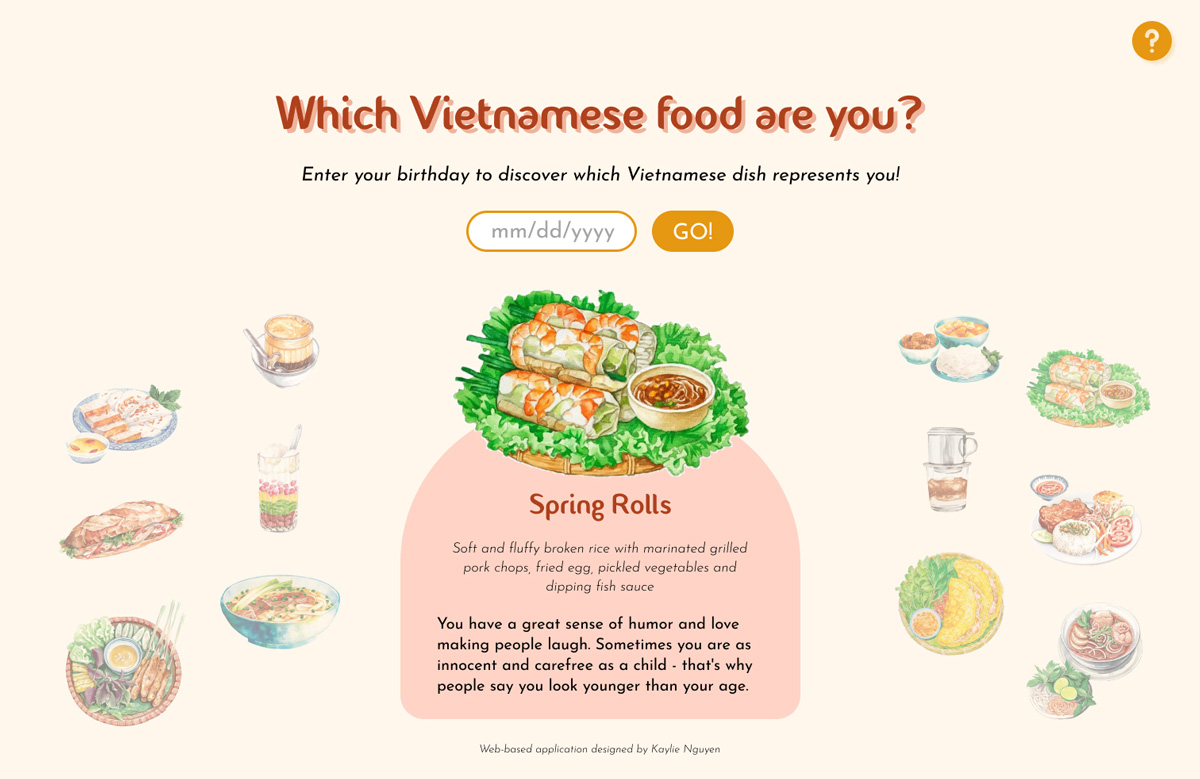
2. Trial and Error: A test of Perseverance
JavaScript took the most time of the project, while the HTML and CSS part is pretty easy and short as this is a one-page web-based application. I was completely new to JavaScript when jumping into the assignment, thus I was struggling at first. I understood the lectures and could get my homeworks well done but I had a hard time applying them to the actual project. It took me some learning curves to figure things out, but looking back on the process and what I learned, it was worth it.
The two main functions of the site are showing information of the corresponding sign (1) when
the users enter their birthdays (function showDishDetail(dish)
), and (2) when they click on the food images on the sub-menu (function showClickedDish(button)
).
It was a trial-and-error process. I first wrote my own code, tested it on the real site, found
bugs using Developer tools, modified my code, and ran it again - repeatedly until things worked
out. It might take me a whole evening just to run a function, which was frustrating at some
point, but I learned a lot along the way: how to organize variables and data properly, what is
the right syntax for a particular function, how to incorporate multiple functions, conditions
and loops in a logical way.
const dishData = [
{
name: "Pho",
intro: "A soup dish consisting of broth, rice noodles, herbs, and sliced meat (usually beef)",
description: "You are friendly and outgoing, known as 'the diplomat' in your friend group.",
image: 'image-asset/pho.png',
sound: 'sounds/pho.wav',
id: "pho",
},
{
name: "Spring rolls",
intro: "A refreshing appetizer made with shrimp, vegetables, herbs, and rice noodles wrapped in rice paper",
description: "You have a great sense of humor and love making people laugh.",
image: 'image-asset/spring-rolls.png',
sound: 'sounds/spring-rolls.wav',
id: "spring-rolls",
}];
I built a "database" to access the food's information more easily
3. Detour or Shortcut?
But sometimes coding is not only about making things run but also making things run in the most
efficient way. For the function of showing dish detail based on the corresponding clicked button,
most of the other students in my class wrote a set of code for each single button, which was 12
similar sets repeated one after one. This was a detour but a safe way to get things done without
putting in too much brainwork. I was going to do the same thing but was challenged by the
question “Is there a faster way to do it?” I had the idea of using loops to avoid repetitive
lines of code but spent quite some time figuring out how to incorporate it. The Array filter()
method is what I ended up with: it loops through the data of foods’ information that I created
beforehand to find the one matching the clicked button. This wasn’t a genius idea or an eureka
moment but I was happy that I made an effort to shorten and optimize my code.
function showClickedDish(button) {
const food = button.id;
const mainDish = dishData.filter(
item => item.id === food
)[0]
dishImage.src = mainDish.image;
dishName.innerText = mainDish.name;
dishIntro.innerText = mainDish.intro;
dishDescrip.innerText = mainDish.description;
playsound(mainDish.sound);
}
I used Array filter()
to avoid redundance.
Note: The site only works on desktop screens.
This first hand experience with front-end development gave me a big picture of what it’s like to build an interactive website from scratch. The process wasn’t entirely easy but I’m proud of making the most out of this learning opportunity and creating a well-designed product. I also identified some of my weaknesses in development after the assignment and I’m currently looking for resources such as books and online courses to sharpen my skills.
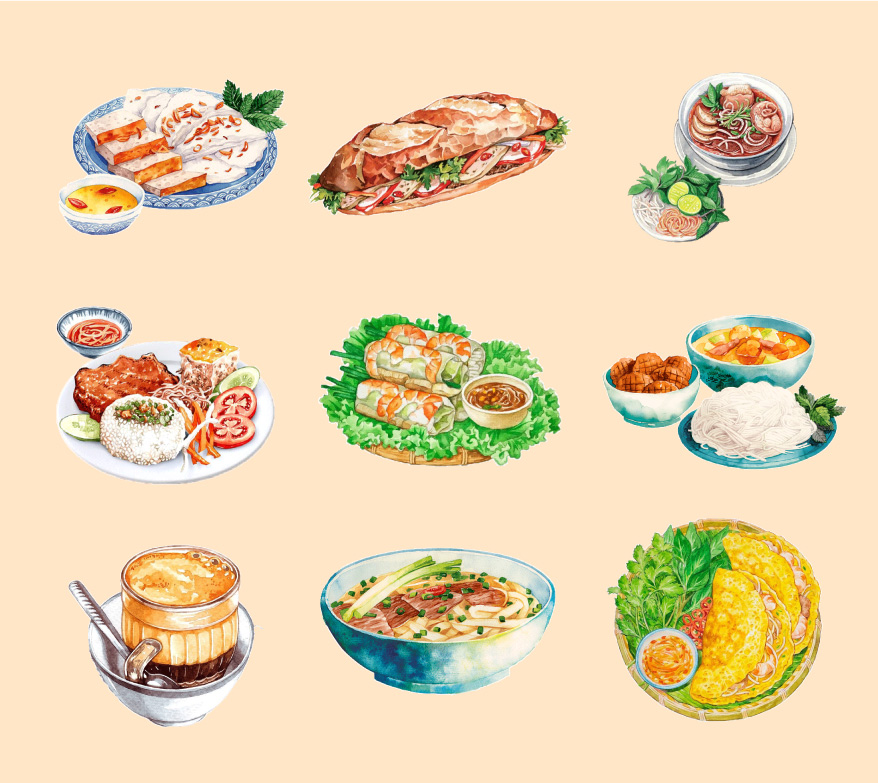